


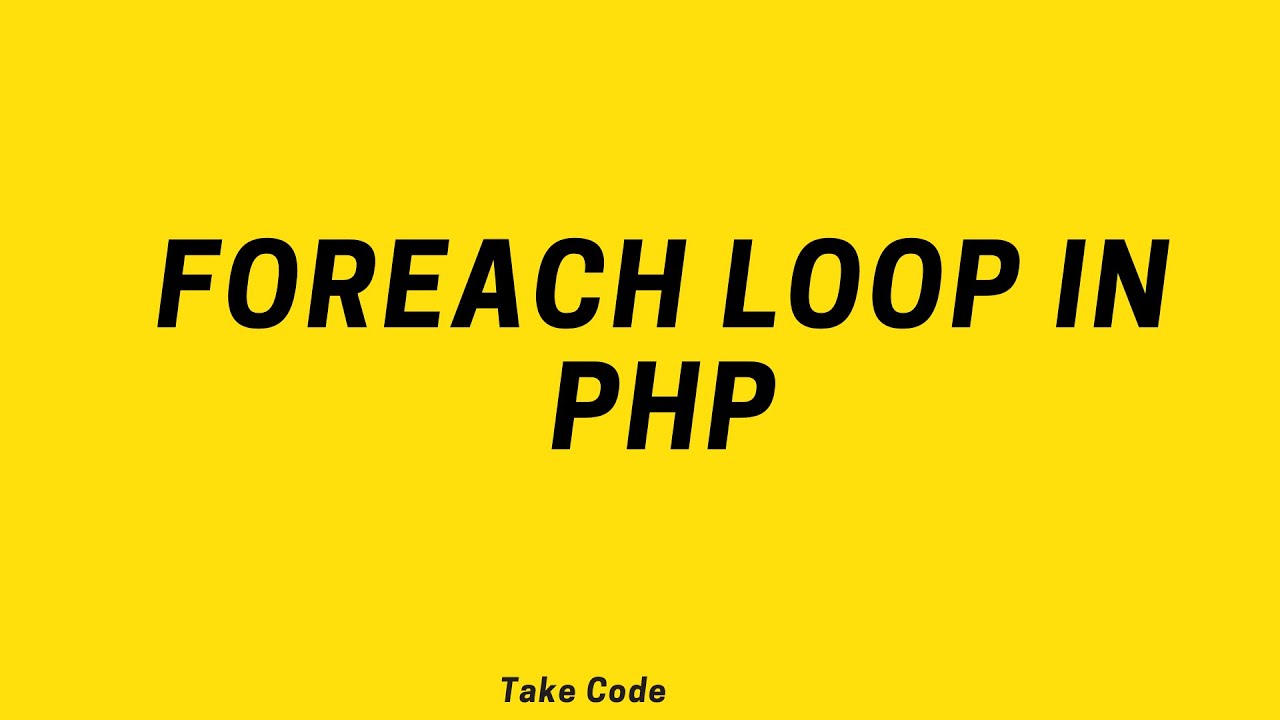
By default array_reverse() will reset all numerical array keys to start counting from zero while literal keys will remain unchanged unless a second parameter preserve_keys is specified as true.To resolve that, since our array only has one element, we could use the following methods to return the first (also the only) element:Įcho array_shift($namesCopy) // output: null if array is emptyĮcho array_pop($namesCopy) // output: null if array is emptyĮcho implode($namesCopy) // output: '' if array is emptyĮcho $names_copy ? '' // output: '' if array index is not set or its value is null However, on the flip side there could potentially be an array offset problem when the input array is empty. The advantage to using this approach is that it doesn't modify the original array. This can be changed however, by specifying the fourth parameter preserve_keys as true. Please note that by default array_slice() reorders and resets the numeric array indices. Next($names) // move array pointer to 2nd elementĮcho reset($namesCopy) // output: "john"Īs you can see from the results, we've obtained the first element of the array without affecting the original.Įcho array_slice($names, 0, 1) // output: "john" If you do not wish to reset the internal pointer of the array, you can work around it by creating a copy of the array simply by assigning it to a new variable before the reset() function is called, like so: This method has a simple syntax and support for older PHP versions:īe careful when resetting the array pointer as each array only has one internal pointer and you may not always want to reset it to point to the start of the array. The PHP reset() function not only resets the internal pointer of the array to the start of the array, but also returns the first array element, or false if the array is empty. Please note that we'll be using the following array for all the examples that follow: In PHP, there are a number of ways you could access the value of the first element of an out-of-order (or non-sequential) array. Get First Element of an Out-Of-Order or Non-Sequential Array However, starting from PHP 7.1, you can specify the specific key you're looking to unpack but we're not covering that since it's not within the scope of this article. $sequentialArray = Īccessing the First Element of Array Directly:Įcho $sequentialArray ? '' // output: 'foo'īy using ? (the null coalescing operator) you can return the first index if it's set and not null, or return an empty string otherwise.įor a non-sequential array this would throw an error because list() tries to unpack array values sequentially (starting from the first index - i.e. Consider for example the following array: It's fairly easy and straightforward to get the first element of a sequential array in PHP.
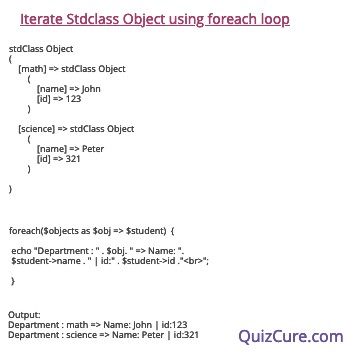
Get First Element of an Array With Sequential Numeric Indexes
